Decision Making (if, if-else, nested if and switch cases in Java)
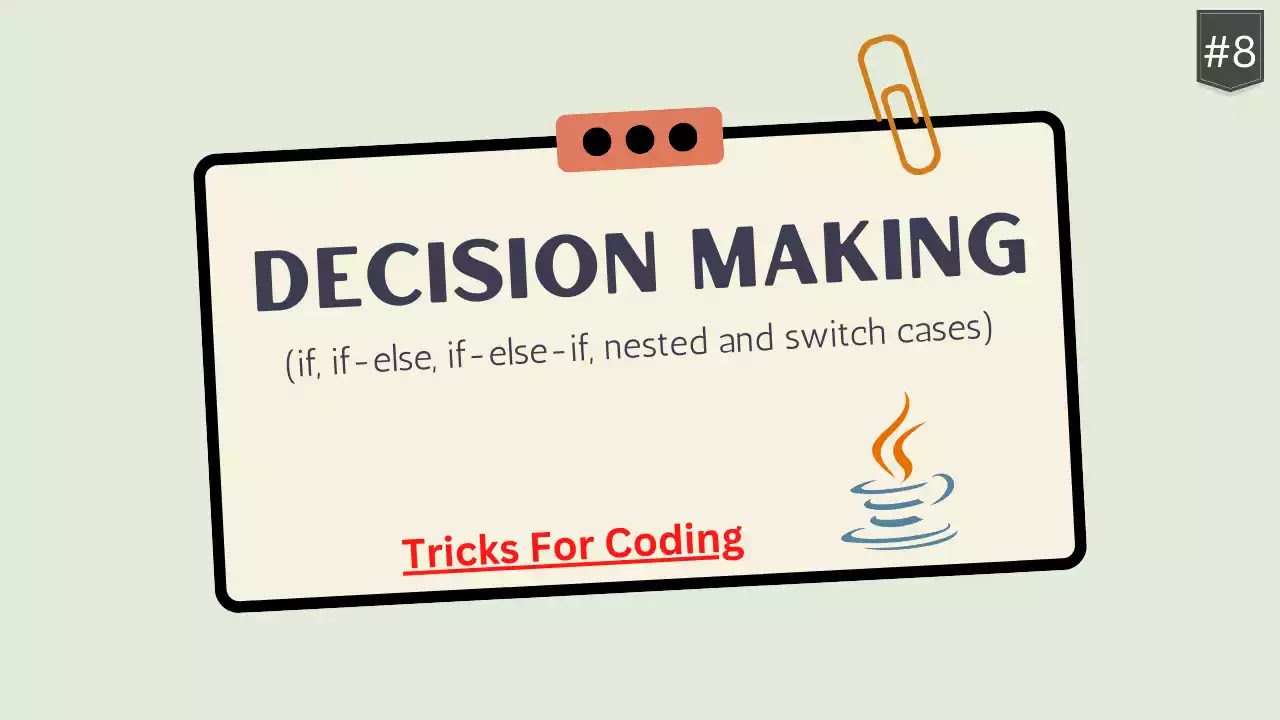_11zon.webp)
Decision Making:
There are two sorts of decision-making constructs in Java. They are:
- if constructs
- switch constructs
The if Statement:
An if constructs comprises of a Boolean outflow emulated by one or more proclamations.The syntax for using this construct is as follows:
if() {
//Statements if the condition is true
}
In the event that the Boolean construct assesses to true, then the scope of code inside the if proclamation will be executed. If not the first set of code after the end of the if construct (after the end wavy prop) will be executed.
Sample Implementation:
The if…else Statement:
An if proclamation can be trailed by a non-compulsory else explanation, which executes when the Boolean outflow is false. The syntax for this construct is as follows:
if("condition"){
//Executes if condition is true
}
else{
//Executes if condition is false
}
Sample Implementation:
public class IfElseExample {
public static void main(String[] args) {
int number=5;
if(number%2==0){
System.out.println("even number");
}else{
System.out.println("odd number");
}
}
}
The if…else if Statement:
An if proclamation can be trailed by a non-compulsory else if…else explanation, which is exceptionally helpful to test different conditions utilizing single if…else if articulation. At the point when utilizing if , else if , else proclamations there are few focuses to remember.
- An if can have zero or one else’s and it must come after any else if’s.
- An if can have zero to numerous else if’s and they must precede the else.
- If one of the if conditions yield a true, the other else ifs and else are ignored.
The syntax for using this decision-making construct Is as follows:
if(condition_1){
//Execute if condition_1 is true
}
else if(condition_2){
//Execute if condition_2 is true
}
else if(condition_3){
//Execute if condition_3 is true
}
else
{
//Execute if all conditions are false
}
Sample Implementation:
Nested if…else Statement:
It is legitimate to home if-else constructs, which implies you can utilize one if or else if proclamation inside an alternate if or else if explanation. The syntax for using this construct Is as follows:
if(condition_1){
//Execute if condition_1 is true
if(condition_2){
//Execute if condition_2 is true
}
}
else if(condition_3){
//Execute if condition_3 is true
}
else
{
//Execute if all conditions are false
}
Sample Implementation:
import java.util.*;
class example
{
public static void main(String args[])
{
//input
int x=50;
int y=60;
//condition1
if(x==50){
//nested condition
if(y==60){
System.out.println("Correct Answer");
}
}
}
}
The switch Statement:
A switch construct permits a variable to be tried for equity against a rundown of values. Each value is known as a case, and the variable being exchanged is checked for each case. The syntax for using this decision-making construct is as follows:
switch(){
case value1:
//Statements
break;
case value2 :
//Statements
break;
default:
//Optional
}
The accompanying runs apply to a switch construct:
- The variable utilized as a part of a switch explanation must be a short, byte, char, or int.
- You can have any number of case explanations inside a switch. Each case is trailed by the value to be contrasted with and a colon
- The value for a case must be the same type as the variable in the switch and it must be a steady or exacting value.
- When the variable being exchanged is equivalent to a case, the announcements after that case will execute until a break is arrived at.
- When a break is arrived at, the switch ends, and the stream of control bounces to the following line after the switch.
- Not each case needs to contain a break. In the event that no break shows up, the stream of control will fall through to consequent cases until a break is arrived at.
- A switch articulation can have a discretionary default case, which must show up toward the end of the switch. The default case can be utilized for performing an undertaking when none of the cases is true. No break is required in the default case. However, as per the convention, the use of the same is recommended.
Samle Implementation:
public class Main {
public static void main(String[] args) {
int day = 7;
switch (day) {
case 1:
System.out.println("Monday");
break;
case 2:
System.out.println("Tuesday");
break;
case 3:
System.out.println("Wednesday");
break;
case 4:
System.out.println("Thursday");
break;
case 5:
System.out.println("Friday");
break;
case 6:
System.out.println("Saturday");
break;
case 7:
System.out.println("Sunday");
break;
}
}
}
Post a Comment